单链表各种操作的代码实现
文章目录
- 单链表各种操作的代码实现
- SList.h:
- SList.c:
- Test.c:
今天简简单单的复习一下单链表的各种操作。
具体操作有:打印,尾插,头插,尾删,头删,在任意结点之前插入,删除任意结点,malloc一个新结点,在所给链表中查找数据x,并返回它的结点等。
标注的比较详细,可以借助注释食用哦。
代码要分为三个项:(全部在一个项中是不规范的)
SList.h:包的引用和函数的声明
SList.c:各个操作的实现
SList.c:各个操作的实现
SList.h:
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
#define _CRT_SECURE_NO_WARNINGS 1
typedef int SLTDataType;
struct SListNode
{
SLTDataType data; //链表的数据
struct SListNode* next; //链表的指针
};
typedef struct SListNode SLTNode;//改个名字
void SListPrint(SLTNode* phead);//打印
void SListPushBack(SLTNode** pphead,SLTDataType);//尾插
void SListPushFront(SLTNode** pphead, SLTDataType x);//头插
void SListPopBack(SLTNode** pphead);//尾删
void SListPopFront(SLTNode** pphead);//头删
void SListInsert(SLTNode** pphead, int pos, SLTDataType x);//在任意位置插入
void SListErase(SLTNode** pphead, int pos);//在任意位置删除
SLTNode* BuySListNode(SLTDataType x);//malloc一个结点
SLTNode* SListFind(SLTNode*phead, SLTDataType x);//在所给链表中查找数据x,并返回它的结点
SList.c:
#define _CRT_SECURE_NO_WARNINGS 1
#include"SList.h"
SLTNode* BuySListNode(SLTDataType x)//malloc一个结点
{
SLTNode* newnode = (SLTNode*)malloc(sizeof(SLTNode));
newnode->data = x;
newnode->next = NULL;
return newnode;
}
void SListPrint(SLTNode* phead)//打印
{
SLTNode* cur = phead;
if (phead != NULL)
{
while (cur != NULL)
{
printf("%d->", cur->data);
cur = cur->next;
}
printf("NULL\n");
}
else
{
printf("您输入的链表为空");
}
}
void SListPushBack(SLTNode** pphead, SLTDataType x)//尾插
{
SLTNode* newnode = BuySListNode(x);
SLTNode* tail = *pphead;
if (*pphead == NULL)
{
*pphead = newnode;
}
else
{
//找尾节点的指针
while (tail->next != NULL)
{
tail = tail->next;
}
tail->next = newnode;
}
}
void SListPushFront(SLTNode** pphead, SLTDataType x)//头插
{
SLTNode* newnode = BuySListNode(x);
newnode->next = *pphead;
*pphead = newnode;
}
void SListPopFront(SLTNode** pphead)//头删
{
if (*pphead == NULL)
return;
else
{
SLTNode* tmp = *pphead;
*pphead = tmp->next;
free(tmp);
}
}
void SListPopBack(SLTNode** pphead)//尾删
{
SLTNode* tmpt = *pphead;
if (*pphead == NULL)
{
return;
}
else if (tmpt->next == NULL)
{
free(*pphead);
*pphead = NULL;//千万不要把==看成=!!
}
else
{
//SLTNode* tmpt2 = tmpt->next;
while (tmpt->next->next != NULL)
{
tmpt = tmpt->next;
}
free(tmpt->next);
tmpt->next = NULL;
}
}
void SListInsert(SLTNode** pphead, SLTNode* pos, SLTDataType x)//在任意结点之前插入数据
{
SLTNode* newnode = BuySListNode(x);
if (*pphead == NULL)
{
*pphead = newnode;
newnode->next = NULL;
}
else if (pos == *pphead)
{
newnode->next = *pphead;
*pphead = newnode;
}
else
{
SLTNode* tmpt = *pphead;
while (tmpt->next != pos)
{
tmpt = tmpt->next;
}
tmpt->next = newnode;
newnode->next = pos;
}
}
SLTNode* SListFind(SLTNode* phead, SLTDataType x)//查找
{
SLTNode* cur = phead;
while (cur)
{
if (cur->data == x)
return cur;
else
cur = cur->next;
}
return NULL;
}
void SListErase(SLTNode** pphead, SLTNode* pos)//删除任意结点
{
SLTNode* cur = *pphead;
if (*pphead == NULL)
{
return;
}
else if(pos==*pphead)
{
*pphead = cur->next;
free(cur);
cur = NULL;
}
else
{
while (cur->next != pos)
{
cur = cur->next;
}
cur->next = pos->next;
free(pos);
}
}
Test.c:
#pragma once
#include"SList.h"
test1()
{
SLTNode* phead = NULL;;
SListPushBack(&phead, 1);
SListPushBack(&phead, 2);
SListPushBack(&phead, 3);
SListPrint(phead);
//想在1前面插入0.
SLTNode* pos = SListFind(phead, 1);//先找到1
if (pos != NULL)
{
SListInsert(&phead, pos, 0);
SListPrint(phead);
}
else
printf("没有找到您要找的位置");
//想要删除链表中的2.
SLTNode* pos2 = SListFind(phead,2);//先找到2
if (pos2 != NULL)
{
SListErase(&phead, pos2);
SListPrint(phead);
}
else
{
printf("没有找到您要删除的元素");
}
}
int main()
{
test1();
return 0;
}
觉得对自己有帮助的小伙伴可以点个赞哦
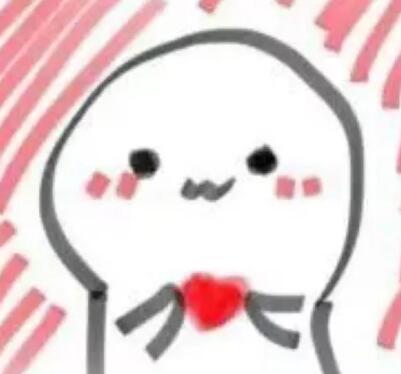