一.作业
1.栈的手写
#include <iostream>using namespace std;// 封装一个栈class stcak{private: int *data; // int max_size; // 最大容量 int top; // 下标public: // 无参构造函数 stcak(); // 有参构造函数 stcak(int size); // 拷贝构造函数 stcak(const stcak &other); // 析构函数 ~stcak(); // 判空函数 bool empty(); // 判满函数 bool full(); // 扩容函数 void resize(int new_size); // 返回元素个数函数 int size(); // 向栈顶插入元素函数 void push(int value); // 删除栈顶元素函数 int pop(); // 访问栈顶元素函数 int get_top(); // 赋值重载函数 stcak &operator=(const stcak &other); // 遍历栈里元素函数 void show();};// 无参构造函数stcak::stcak() : max_size(10){ data = new int[10]; max_size = 10; top = -1; cout << "无参构造" << endl;}// 有参构造函数stcak::stcak(int size){ data = new int[size]; max_size = size; top = -1; cout << "有参构造" << endl;}// 拷贝构造函数stcak::stcak(const stcak &other){ max_size = other.max_size; top = other.top; data = new int[max_size]; for (int i = 0; i <= top; i++) { data[i] = other.data[i]; } cout << "拷贝构造" << endl;}// 析构函数stcak::~stcak(){ delete[] data; cout << "析构函数" << endl;}// 判空函数bool stcak::empty(){ return top == -1;}// 判满函数bool stcak::full(){ return top == max_size - 1;}// 扩容函数void stcak::resize(int new_size){ int *new_data = new int[new_size]; for (int i = 0; i <= top; i++) { new_data[i] = data[i]; } delete[] data; data = new_data; max_size = new_size;}// 返回元素个数函数int stcak::size(){ return top + 1;}// 向栈顶插入元素函数void stcak::push(int value){ if (full()) { // 调用扩容函数 resize(max_size * 2); } data[++top] = value;}// 删除栈顶元素函数int stcak::pop(){ if (empty()) { cout << "栈是空的"; return -1; } return data[top--]; // 出栈}// 访问栈顶元素函数int stcak::get_top(){ if (empty()) { cout << "栈是空的"; return -1; } return data[top]; // 出栈}// 赋值重载函数stcak &stcak::operator=(const stcak &other){ if (this == &other) { return *this; } delete[] data; max_size = other.max_size; top = other.top; data = new int[max_size]; for (int i = 0; i <= top; i++) { data[i] = other.data[i]; } return *this;}// 遍历栈里元素函数void stcak::show(){ if (empty()) { cout << "栈是空的"; return; } cout << "栈里元素有:"<<endl; for (int i = 0; i <= top; i++) { cout<< data[i] <<'\t'; } cout <<endl;}/******************主函数*********************/ int main(){ stcak s1(20); cout << s1.size() << endl; s1.push(1); s1.push(2); s1.show(); cout << s1.size() << endl; stcak s2 = s1; return 0;}
效果图:
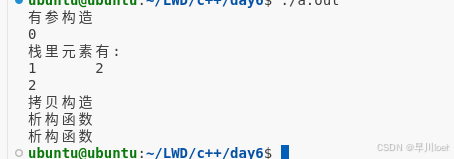
2.队列的手写
#include <iostream>using namespace std;class queue{private: int *data; // 容器 int max_size; // 最大容量 int front; // 头下标 int tail; // 尾下标public: // 无参构造函数 queue(); // 有参构造函数 queue(int size); // 拷贝构造函数 queue(const queue &other); // 析构函数 ~queue(); // 判空函数 bool empty(); // 判满函数 bool full(); // 扩容函数 void resize(int new_size); // 元素个数函数 int size(); // 向队列尾部插入元素函数 void push(int value); // 删除首个元素函数 出队 int pop(); // 遍历队列元素 void show(); // 赋值重载函数 queue &operator=(const queue &other);};// 无参构造函数queue::queue() : max_size(10){ data = new int[10]; max_size = 10; front = tail = -1; cout << "无参构造" << endl;}// 有参构造函数queue::queue(int size){ data = new int[size]; max_size = size; front = tail = 0; cout << "有参构造" << endl;}// 拷贝构造函数queue::queue(const queue &other){ max_size=other.max_size; front=other.front; tail=other.tail; data=new int[max_size]; for (int i = front; i != tail; i = (i + 1) % max_size) { data[i]=other.data[i]; } cout << "拷贝构造" << endl;}// 析构函数queue::~queue(){ delete[] data; cout << "析构函数" << endl;}// 判空函数bool queue::empty(){ return front == tail;}// 判满函数bool queue::full(){ return (tail + 1) % max_size == front;}// 元素个数函数int queue::size(){ return (tail - front + max_size) % max_size;}// 扩容函数void queue::resize(int new_size){ int *new_data = new int[new_size]; for (int i = front; i <= tail; i++) { new_data[i] = data[i]; } data = new_data; max_size = new_size;}// 向队列尾部插入元素函数void queue::push(int value){ if (full()) { // 调用扩容函数 resize(max_size * 2); } data[tail] = value; tail = (tail + 1) % max_size;}// 删除首个元素函数 出队int queue::pop(){ if (empty()) { cout << "队列为空" << endl; return -1; } cout << data[front] << "出队" << endl; front = (front + 1) % max_size; return 0;}// 遍历队列元素void queue::show(){ if (empty()) { cout << "队列为空" << endl; return; } cout << "队列元素:" << endl; for (int i = front; i != tail; i = (i + 1) % max_size) { cout << data[i] << '\t'; } cout << endl;}// 赋值重载函数queue &queue::operator=(const queue &other){ if (this == &other) { return *this; } delete []data; max_size=other.max_size; front=other.front; tail=other.tail; data=new int[max_size]; for (int i = front; i != tail; i = (i + 1) % max_size) { data[i]=other.data[i]; } cout << "拷贝赋值函数" <<endl; return *this; }/******************主函数*********************/int main(){ queue s1(20); s1.push(1); s1.push(2); s1.show(); // s1.pop(); // s1.pop(); // s1.show(); queue s2=s1; queue s3; s3=s2; return 0;}
效果图:
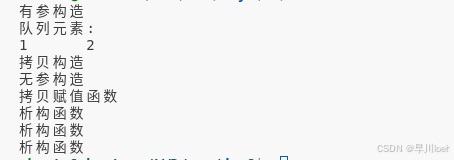
二.思维导图
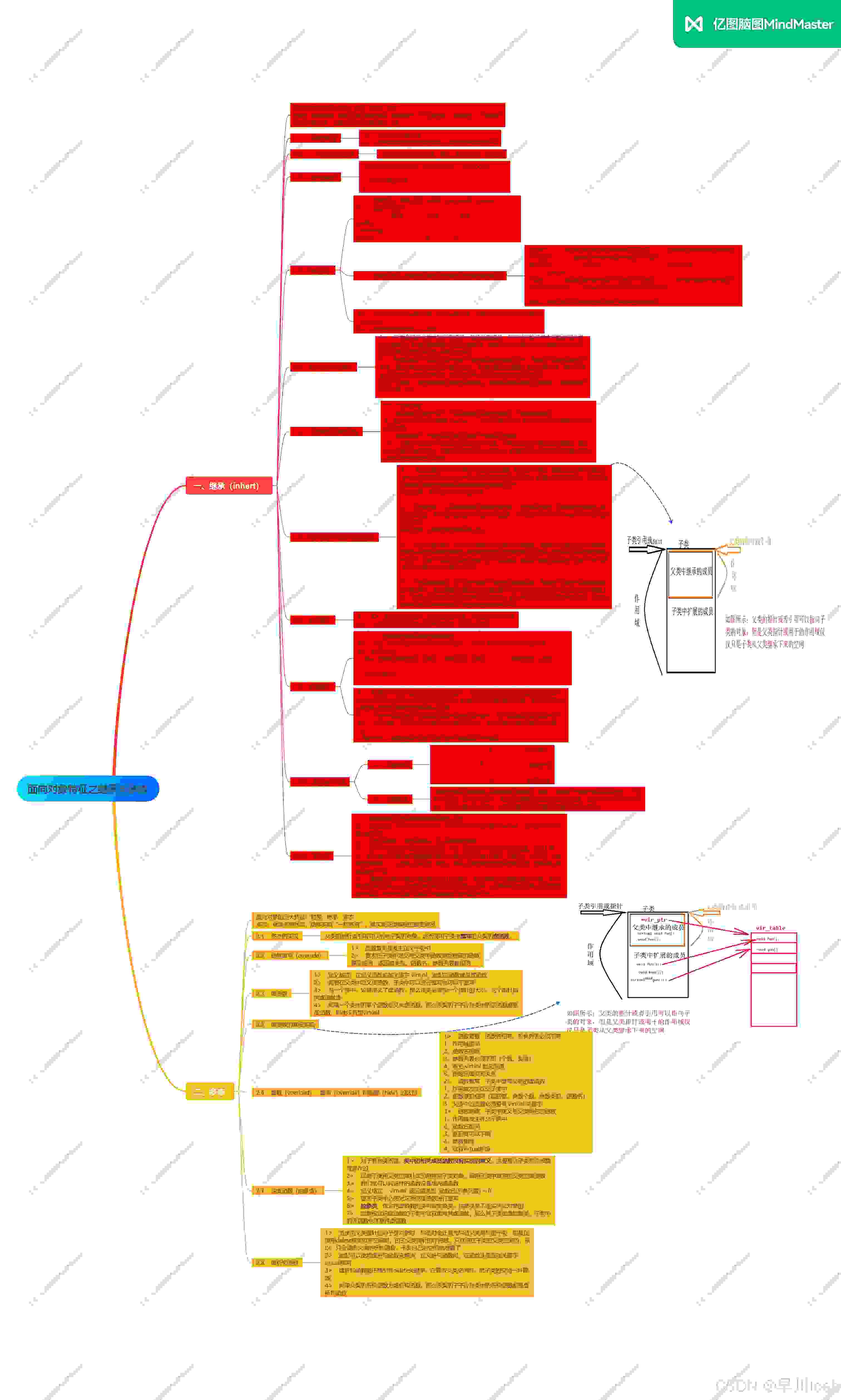